How to Access and Use the Claude API

Maxwell Timothy
Apr 11, 2025
10 min read
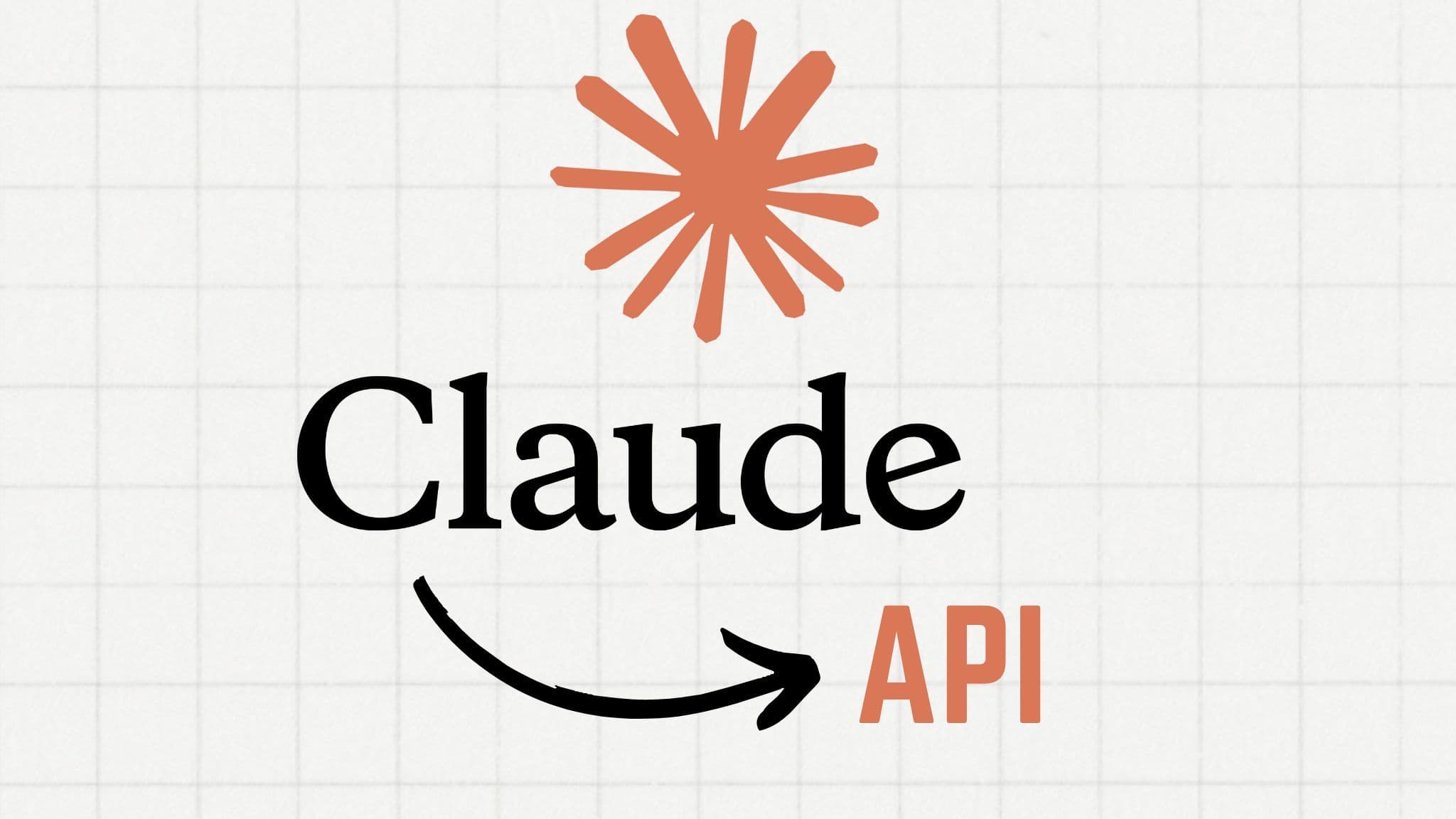
Claude has quickly become one of the most reliable models in the AI space. Built by Anthropic, it's designed with a strong focus on safety, reasoning ability, and natural language understanding.
But beyond the tech specs, what makes Claude genuinely stand out is how efficient and easy it is to use in real-world applications. Whether you're building a customer support bot, summarizing documents, or doing structured reasoning at scale, Claude's models offer a clean balance between power and usability.
And it's not just one model. Claude comes in several versions—each one tuned for different needs. There's Claude 3.5 Sonnet, a solid choice. Then you’ve got Claude 3.7 Sonnet as well. Both optimized for faster outputs and edge deployments. Each version gives you more control, more speed, or more reasoning power depending on the project you're building.
In this guide, we’ll walk through exactly how you can integrate Claude into your own product or workflow. We’ll be using Claude 3.5 Sonnet in our example, but the process is very similar across all Claude models.
Connecting to the Anthropic API
To start using Claude 3.5 Sonnet in your application, you'll need to first connect to the Anthropic API. Below, we’ll walk through how to get set up and get your environment ready for integration. We'll also provide you with a detailed overview of the key components of the API.
Step 1: Register and Get API Access
The first thing you need to do is sign up for an account with Anthropic.
- Visit the Anthropic Console and create an account by providing your basic details (name, email, etc.).
- After signing up, you’ll need to verify your email address to complete the registration process.
Once your account is set up, you’ll be ready to move on to the next step.
Step 2: Obtain Your API Key
To interact with the Claude 3.5 Sonnet API, you will need an API key. Here’s how you can get it:
- Head to the API Keys section in the Anthropic Console.
- Click on Create Key to generate a new API key.
Important: This key is essential for authenticating your requests to the API, so make sure to store it securely.
Step 3: Set Up Your Development Environment
With the API key ready, it’s time to set up your development environment. For simplicity, we’ll be using Python in this example, but you can integrate the API with any language that supports HTTP requests.
Install the Anthropic Python Library
You need to install the Anthropic library to interact with the API. Here’s the command to install it using pip:
pip install anthropic
Once the library is installed, you can initialize the API client by inserting your API key into the following code:
import
1import anthropic23# Create an instance of the Anthropic API client45client = anthropic.Anthropic(api_key='your_api_key_here')
With this setup, you can now start sending requests to the Claude 3.5 Sonnet model.
Step 4: Testing the Connection
Before diving into specific API functionality, let’s test the connection to make sure everything is working. You can do this by sending a simple message query to the Claude 3.5 Sonnet model.
Here’s an example of a basic message request:
test connection
1response = client.messages.create(23model="claude-3-5-sonnet-20240620",45messages=[{"role": "user", "content": "Hello, Claude!"}]67)89print(response)
If everything is set up properly, you should get a response from the API. This ensures that your connection to the Claude 3.5 Sonnet model is working.
Claude 3.5 Sonnet API: Messages API vs. Text Completion API
The Claude 3.5 Sonnet API offers two main ways of interacting with the model: the Messages API and the older Text Completions API.
- Messages API: This is the modern, preferred method for interacting with the model. It enables dynamic, multi-turn conversations with more complex behaviors.
- Text Completions API: This is a legacy feature that supports basic text generation, but lacks the flexibility and power of the Messages API.
We strongly recommend using the Messages API moving forward for better support and enhanced functionality.
Additionally, it’s important to note that Artifacts (such as content generated during conversations) are not accessible through the API directly. To interact with Artifacts, you’ll need to use Claude.ai’s web interface, which allows you to edit and download content easily.
Working with the Messages API
The Messages API is the core functionality of the Claude 3.5 Sonnet model. It allows you to send a list of input messages (either text or images) and receive generated responses.
Required Parameters
The key components of a message request include:
- model: The model you want to use (e.g., "claude-3-5-sonnet-20240620").
- messages: A list of messages to send. Each message must have a role (either "user" or "assistant") and content (the text content of the message).
For example:
messages api
1response = client.messages.create(23model="claude-3-5-sonnet-20240620",45messages=[67{"role": "user", "content": "How are you today?"},89{"role": "assistant", "content": "I'm doing great, thank you!"}1011]1213)1415print(response)16
Optional Parameters
There are several optional parameters that you can include in your requests to control the behavior of the model:
- max_tokens: Controls the maximum number of tokens (words or characters) in the model’s response.
- temperature: Affects the randomness of the output. Higher values (e.g., 0.8) generate more varied responses, while lower values (e.g., 0.2) make the output more deterministic.
- stop_sequences: Defines custom text sequences that signal the model to stop generating further output.
- stream: If set to True, the response is streamed incrementally.
- system: A system prompt to provide additional context or instructions to the model.
- tools: Defines specific tools that the model may use (e.g., for querying a database).
- top_k: Limits the model’s token selection to the top K options, which can help remove unlikely responses.
- top_p: Uses nucleus sampling to only consider tokens within a specified cumulative probability.
These optional parameters help fine-tune how Claude 3.5 Sonnet generates its responses, allowing you to control various aspects of the output.
Now that your environment is set up and you have a working connection to the Claude 3.5 Sonnet API, you’re ready to start building more complex interactions with the model. Whether you're integrating it into a chatbot, customer service tool, or any other application, the next step is to explore how to manage multiple-turn conversations and enhance the richness of the interactions.
Using System Prompts and Stop Sequences for Tailored Responses
This section explains how you can leverage system prompts and stop sequences to guide and control Claude 3.5 Sonnet’s responses, ensuring they meet your specific needs. These tools provide a way to set the tone of the conversation, control response length, and provide additional context that shapes how the model responds.
System Prompts: Shaping the Model's Behavior
System prompts allow you to inject context into the model, influencing how it behaves during interactions. This is particularly useful when you need the model to maintain a certain tone or persona throughout the conversation.
For instance, imagine you're building a chatbot for a customer support service, and you want it to always be polite, clear, and professional. Here’s how you can set that up:
system
1response = client.messages.create(23model="claude-3-5-sonnet-20240620",45max_tokens=1024,67messages=[{"role": "user", "content": "How can I track my order?"}],89system="You are a friendly and professional customer support agent who provides clear instructions.",1011stop_sequences=["Thank you for contacting us."],1213temperature=0.71415)1617
In this example:
- The system prompt sets the tone, asking the model to behave like a polite, professional customer support agent.
- The stop_sequences parameter ensures the conversation ends at "Thank you for contacting us," giving a structured and courteous wrap-up to the conversation.
System prompts allow you to control the model’s tone, ensuring it is suited to the specific context of your application, whether that's customer support, technical assistance, or any other area where specific behavioral guidelines are necessary.
Stop Sequences: Controlling the Output Flow
Stop sequences define specific phrases or tokens where the model should stop generating additional content. This is useful when you want to control how much the model responds, avoiding responses that are too lengthy or off-topic.
Let’s say you're building a chatbot for troubleshooting, and you want the model to stop after delivering a solution to a specific issue, such as a Wi-Fi connectivity problem:
stop sequence
123response = client.messages.create(45model="claude-3-5-sonnet-20240620",67max_tokens=1024,89messages=[{"role": "user", "content": "I can't connect to the Wi-Fi, what should I do?"}],1011system="You are a helpful technical support assistant with clear, step-by-step guidance.",1213stop_sequences=["If the problem persists, please contact support."],1415temperature=0.61617)
Here:
- The system prompt guides the model to offer clear, actionable steps for resolving technical issues.
- The stop_sequences ensures that once the model provides troubleshooting instructions and ends with "If the problem persists, please contact support," the conversation is neatly concluded.
Stop sequences help ensure that responses stay focused and concise, providing a satisfying conclusion without the risk of the model continuing to generate irrelevant or excessive content.
Tool Definitions: Extending Claude 3.5 Sonnet’s Capabilities
Claude 3.5 Sonnet's ability to integrate with external tools can enhance its functionality, allowing it to perform actions like querying a database, processing user inputs dynamically, or interacting with third-party services.
For example, let’s say you want to create a chatbot that helps users book appointments. You can define a tool to check available appointment slots and book them for the user:
tools
1tools = [2{3"name": "check_appointments",4"description": "Check available appointment slots for a given service and time.",5"input_schema": {6"type": "object",7"properties": {8"service": {9"type": "string",10"description": "The type of service the user wants to book (e.g., dentist, haircut)."11},12"date": {13"type": "string",14"description": "The preferred date for the appointment (e.g., '2025-05-01')."15}16},17"required": ["service", "date"]18}19}20]21#Once you’ve defined the tool, you can use it to check availability and book appointments:22response = client.messages.create(23model="claude-3-5-sonnet-20240620",24messages=[{"role": "user", "content": "I need to book a dentist appointment for May 1st."}],25tools=tools26)
In this example:
- The check_appointments tool allows the model to query available time slots for a specific service (like a dentist appointment).
- Based on the user’s request, the model will use the tool to retrieve available times and help the user book the appointment.
By defining tools like this, you can extend Claude 3.5 Sonnet’s functionality to handle specific tasks, such as checking appointment availability, retrieving product information, or processing user requests in real-time.
Simplify Claude Deployment with Chatbase
If you're looking to easily create a Claude-powered conversational AI tool for your website or business, Chatbase is the perfect solution. With Chatbase, you can quickly upload your documents and train a Claude-powered chatbot—no setup hassle required.
Powered by Claude 3.5 Sonnet, Claude 3.7 Sonnet, Claude 3 Opus, and Claude 3 Haiku, Chatbase gives you easy access to some of the most powerful Claude models available.
How It Works
- Upload Your Document: Add the content your chatbot will use.
- Train Your AI: Chatbase automatically trains your chatbot.
- Deploy: Once trained, you can easily deploy your chatbot into your website or app.
Why Chatbase?
Chatbase makes it easy to build, train, and deploy Claude-powered chatbots with flexibility and minimal effort. Select your model, customize, and get your conversational AI tool up and running in no time.
Start building today—sign up for Chatbase and get started!
Share this article: